Python is a general-purpose, interpreted, high-level programming language with dynamic semantics. Its high-level built-in data structures that combined with dynamic typing and dynamic binding, make it very attractive for Rapid Application Development as well as for use as a scripting language to connect existing components together. It’s simple and easy to learn syntax emphasizes readability and reduces the cost of program maintenance. It also supports modules and packages that encourage program modularity and code reuse. The Python extensive standard library and interpreter are available in source or binary form without charge for all major platforms and can be freely distributed. Let’s discuss popular Python Programs that every Python programmer must know about.
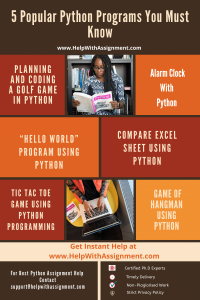
If you are a beginner or writing an assignment on Python programming, here 5 Popular Python Programs you must know.
Top 5 Python Programs Every Python Programming Beginner Should Know About
1. “Hello World” Program Using Python
In Python: Defining Main at the start and invoking main at the end important
Write a Python program that uses a for-loop to print Hello World five times
The output should look like:
Hello World
Hello World
Hello World
Hello World
Hello World
Solution:
Here is code:
def main():
for i in range(0, 5):
print(“Hello World”)
main()
Output:
If you want to learn more about Programming, learn from our Programming Assignment Help experts.
2. Game of Hangman Using Python Program
Computing Using Python: Write a program that plays the game of hangman. Use characters to print the hangman’s status. Triple-quoted strings will be useful. Hint: draw the entire hangman status as a string picture with a full picture for each partial hangman status.
Solution:
import random
board = [
‘ +—+ n | | n | n | n | n | n========= n’,
‘ +—+ n | | n 0 | n | n | n | n========= n’,
‘ +—+ n | | n 0 | n | | n | n | n========= n’,
‘ +—+ n | | n 0 | n /| | n | n | n========= n’,
‘ +—+ n | | n 0 | n /| | n | n | n========= n’,
‘ +—+ n | | n 0 | n /| | n / | n | n========= n’,
‘ +—+ n | | n 0 | n /| | n / | n | n========= n’
]
class Hangman:
def __init__(self,word):
self.word = word
self.missed_letters = []
self.guessed_letters = []
def guess(self,letter):
if letter in self.word and letter not in self.guessed_letters:
self.guessed_letters.append(letter)
elif letter not in self.word and letter not in self.missed_letters:
self.missed_letters.append(letter)
else:
return False
return True
def hangman_over(self):
return self.hangman_won() or (len(self.missed_letters) == 6)
def hangman_won(self):
if ‘_’ not in self.hide_word():
return True
return False
def hide_word(self):
rtn = ”
for letter in self.word:
if letter not in self.guessed_letters:
rtn += ‘_’
else:
rtn += letter
return rtn
def print_game_status(self):
print board[len(self.missed_letters)]
print ‘Word: ‘ + self.hide_word()
print ‘Letters Missed: ‘,
for letter in self.missed_letters:
print letter,
print
print ‘Letters Guessed: ‘,
for letter in self.guessed_letters:
print letter,
print
def rand_word():
bank = [‘the’,‘living’,‘pearl’,‘.com’,‘captain’,‘deadbones’]
return bank[random.randint(0,len(bank))]
def main():
game = Hangman(rand_word())
while not game.hangman_over():
game.print_game_status()
user_input = raw_input(‘nEnter a letter: ‘)
game.guess(user_input)
game.print_game_status()
if game.hangman_won():
print ‘nCongratulations! You are the winner of Hangman!’
else:
print ‘nSorry, you have lost in the game of Hangman…’
print ‘The word was ‘ + game.word
print ‘nGoodbye!n’
if __name__ == “__main__”:
main()
3. Planning and Coding a Golf Game in Python
Question
You are to plan and then code a golf game program in Python 3, as described in the following information and sample output. Use simple functions, selection, and repetition. However, it is important to note that you are not to define any of your own classes or use lists or dictionaries.
Program Features:
Ensure that your program has the following features, as demonstrated in the sample output below:
- a welcome message with your name in it
- a menu for the user to view the instructions, play the game or quit
- a play game option (details below)
- the program is to return to the menu and loop until the user chooses to quit
- all inputs should be error-checked by the program as demonstrated in the sample output Playing the game:
- For each swing, the player chooses a club and then the program generates the distance hit for each shot, updating the distance to the hole accordingly. Play proceeds until the ball is in the hole (distance to the hole is zero), and then the program informs the user of their score.
- The player has three clubs to choose from for each shot. Each club hits the ball a set average distance, but the actual distance randomly varies between 80% and 120% of the average. The clubs and their average distances are:
- Driver: 100m (actual distance will be a random number between 80 and 120)
- Iron: 30m
- Putter: 10m* *When the ball is inside 10m and the putter is used, the shot will be between 80% and 120% of the distance to the hole, not the club’s average distance. The minimum distance the putter can hit is 1m (no 0m hits). All distances are whole numbers.
- If an invalid club is chosen, this is counted as an air swing and the number of shots increases by one, but the ball doesn’t move.
- The ball cannot be a negative distance to the hole. Whether it is in front of or behind the hole, it is still a positive distance to the hole. Python has an abs (absolute value) function that you can use to help with this.
- The score is the number of shots taken to get the ball in the hole. The final output shows the number of shots taken and how this relates to par. Less than 5 (par for this hole) is “under par”, equal to 5 is called “par”, and more than 5 is “over par”. See sample output for exact outputs
Planning:
Write up the algorithm in pseudocode – first! Please do this in a docstring (comment) at the top of your code file after your name, date and brief program details. Follow the guide to good pseudocode and examples presented in the subject to ensure this is done to a high standard.
Solution: https://www.helpwithassignment.com/Solution-Library/plan-and-code-a-golf-game-in-python
4. Tic Tac Toe Game Using Python Programming
Question: Tic tac toe game using python programming language
Solution:
Program –
“”” block array to store the position “””
block = []
for pos in range (0, 9) :
block.append(str(pos + 1))
“”” intialize variable “””
firstPlayerTurn = True
playerWinner = False
“”” function to display board “””
def displayBoard() :
print( ‘n —–‘)
print( ‘|’ + block[0] + ‘|’ + block[1] + ‘|’ + block[2] + ‘|’)
print( ‘ —–‘)
print( ‘|’ + block[3] + ‘|’ + block[4] + ‘|’ + block[5] + ‘|’)
print( ‘ —–‘)
print( ‘|’ + block[6] + ‘|’ + block[7] + ‘|’ + block[8] + ‘|’)
print( ‘ —–n’)
“”” while loop till no player win “””
while not playerWinner :
displayBoard()
if firstPlayerTurn :
print( “Player 1:”)
else :
print( “Player 2:”)
try:
choice = int(input(“>> “))
except:
print(“please enter a valid field”)
continue
“”” if user enter wrong move “””
if block[choice – 1] == ‘X’ or block [choice-1] == ‘O’:
print(“illegal move, plase try again”)
continue
if firstPlayerTurn :
block[choice – 1] = ‘X’
else :
block[choice – 1] = ‘O’
firstPlayerTurn = not firstPlayerTurn
for pos in range (0, 3) :
y = pos * 3
if (block[y] == block[(y + 1)] and block[y] == block[(y + 2)]) :
playerWinner = True
displayBoard()
if (block[pos] == block[(pos + 3)] and block[pos] == block[(pos + 6)]) :
playerWinner = True
displayBoard()
if((block[0] == block[4] and block[0] == block[8]) or
(block[2] == block[4] and block[4] == block[6])) :
playerWinner = True
displayBoard()
“”” print the player who win “””
print (“Player ” + str(int(firstPlayerTurn + 1)) + ” wins!n”)
For screenshots, click here
5. Compare Excel Sheet Using Python Programming
Using python programming language, compare the below excel sheets and print out what’s the same and different in both sheets.
excel file 1:
Name |
Path |
|
python-excel |
users/python/excel |
python-excel1 |
users/python/excel1 |
python-excel2 |
users/python/excel2 |
python-excel3 |
users/python/excel3 |
python-excel4 |
users/python/excel4 |
excel file2:
Name |
Path |
python-excel |
users/python/excel |
python-excel1 |
users/python/excel1 |
python-excel9 |
users/python/excel9 |
python-excel3 |
users/python/excel3 |
python-excel4 |
users/python/excel4 |
Solution:
def print_difference_of_excel_files(File1,File2):
#read full excel1 file
file1=open(File1,’r’)
excel1_content=set(file1.readlines())
file1.close()
# print(excel1_content)
#read full excel2 file
file2=open(File2,’r’)
excel2_content=set(file2.readlines())
file2.close()
#print(excel2_content)
#print difference of two sets for differences
print(‘nn differences nn’)
for line in excel1_content-excel2_content:
print(line)
for line in excel2_content-excel1_content:
print(line)
#print intersection of two sets for same things
print(‘nn same things nn’)
for line in set(excel1_content).intersection(excel2_content):
print(line)
print_difference_of_excel_files(“excel1.xlsx”,”excel2.xlsx”)
To know more about Python programming and Python programming assignment writing, visit our Python Assignment Help page. And if you are a college student and looking for professional assistance in writing your Python Programming assignment, here is how you can get the best assistance for your Python Programming assignments.
We deliver a well-researched academic paper tailored to your specifications at a fair price while ensuring timely delivery. Our service is known for providing top-scoring, plagiarism-free research papers. Additionally, we offer unlimited revisions and 24/7 customer support as part of our commitment to quality.
So, don’t hesitate—place your order today and receive expert academic assistance instantly!
Get Python Programming Assignment Help Now
Fill up the assignment help request form on the right or drop us an email at support@helpwithassignment.com. Feel free to contact our customer support on the company 24/7 Live chat or call us on 312-224-1615.
References:
https://introcs.cs.princeton.edu/python/home/